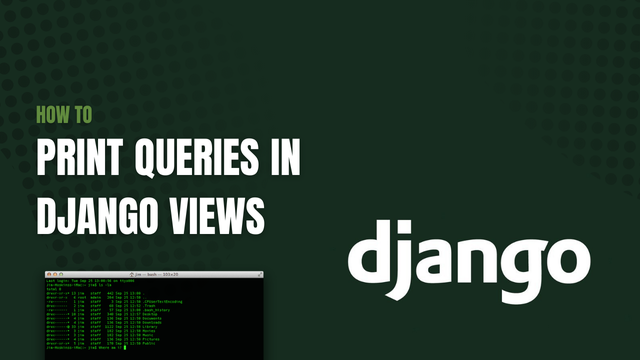
How to Print Queries in Django Views
If you are building a web application with Django you are well aware that the database is an integral part of your application. The need to analyze queries and to then query your data in the most optimal way is at the for front of your development process.
Django provides a handful of tools to do so, some even comes with an extended feature-set as third-party packages with extra dependencies. However, sometimes it's better to keep it simple and just print queries as they happen to get a better understanding of what is being fetched from your database, with minimal effort.
Printing queries within a view allows you to quickly analyze data from particular URLs as you navigate to them.
Follow the steps below to learn how to print queries as SQL in your Django views.
1. Import django.db.connection
Import connection from django.db in your views.py file.
from django.db import connection
2. Use the Print() Statement in a View
def product_detail(request, slug):
print(connection.queries)
product = get_object_or_404(Product, slug=slug)
return render(request, template, {
'product': product,
})
Visit the URL associated with the View
When you have added the print statement to your view you can visit the URL that utilize the view and get your queries displayed in the command line.
[08/Aug/2022 20:58:27] "GET / HTTP/1.1" 500 372543
[{'sql': 'SELECT COUNT(*) AS "__count" FROM "catalog_product" WHERE "catalog_product"."is_published"', 'time': '0.001'}]
Of course there are many other ways to get query information with Django, the most common one being using the django-debug-toolbar. However, at times this can be more complicated and requires you to install external dependencies. This method allows you to quickly analyze queries on a per-view-basis.
