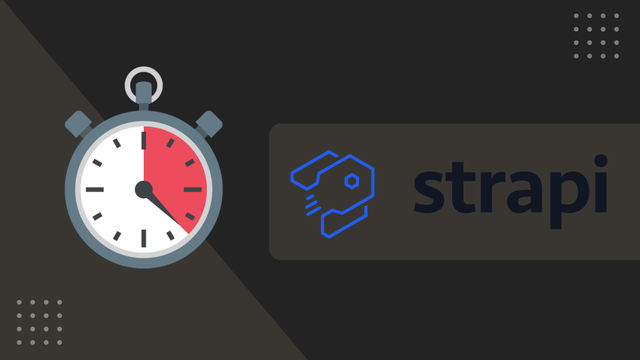
Enable & Write Cron Jobs for Strapi
A cron job is a useful utility that can be found in any unix-like operating system. It can be used to schedule time-based commands without human intervention. With Strapi, we can use cron jobs to import data or run application tasks automatically.
To write cron jobs in Strapi is super simple! In this tutorial I will show you how you can enable and write cron jobs for Strapi.
1. Enable Cron Jobs for Strapi
To write cron jobs in Strapi, we first need to enable them. In earlier versions of Strapi this was done via admin dashboard, however this is no longer the case. In the later versions of Strapi we have to edit the config/server.js file in our project.
// config/server.js
cron: { enabled: true }
A more complete example might look like this.
// config/server.js
module.exports = ({ env }) => ({
host: env('HOST', '0.0.0.0'),
port: env.int('PORT', 1337),
admin: {
auth: {
secret: env('ADMIN_JWT_SECRET', 'SomeSecretKey'),
},
},
cron: { enabled: true },
});
2. Write Cron Jobs for Strapi
Cron jobs in Strapi are written in the config/functions/cron.js. Below you have an example of how to create a cron job and console.log it to your terminal.
// config/functions/cron.js
'use strict';
/**
* Cron config that gives you an opportunity
* to run scheduled jobs.
*
* The cron format consists of:
* [SECOND (optional)] [MINUTE] [HOUR] [DAY OF MONTH] [MONTH OF YEAR] [DAY OF WEEK]
*
* See more details here: https://strapi.io/documentation/v3.x/concepts/configurations.html#cron-tasks
*/
module.exports = {
'*/1 * * * *': async() => {
console.log("I am a cron job and I ran " + new Date());
}
};
3. Understand the Cron Job Format
To write timely cron jobs you need to have a basic understanding how the cron format works. Below you can find a diagram with the format laid out.
* * * * * *
┬ ┬ ┬ ┬ ┬ ┬
│ │ │ │ │ |
│ │ │ │ │ └ day of week (0 - 7) (0 or 7 is Sun)
│ │ │ │ └───── month (1 - 12)
│ │ │ └────────── day of month (1 - 31)
│ │ └─────────────── hour (0 - 23)
│ └──────────────────── minute (0 - 59)
└───────────────────────── second (0 - 59, OPTIONAL)
I hope this tutorial helped you get up and running with cron jobs for Strapi, and hopefully automate some of your repetitive tasks. I have also written a few other Strapi tutorials that you might find interesting.
