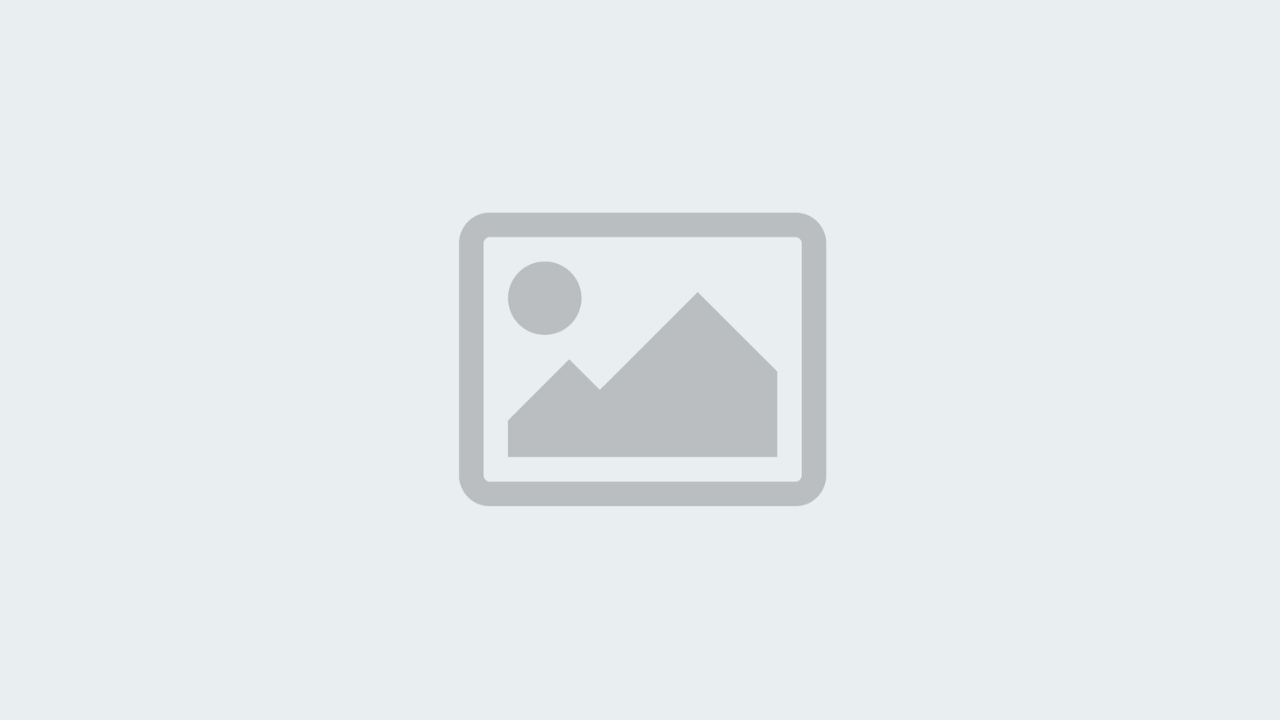
Add Google Analytics Tracking to Your Svelte/Sapper Application
Svelte is a fantastic JavaScript framework (compiler) that let's you build leaner frontends quicker. Svelte also comes with a SSR compatible framework called Sapper. Think of this as your Next.js for React or your Nuxt.js for Vue.js.
Today we are going to add Google Analytics tracking to a Sapper application. We are going to use Svelte's reactive statement $: to achieve tracking both for server-side and client-side.
Add Google Analytics Tracking Code to Your Project
We start by adding our analytics tracking code to our <head></head>
in our template.html file in our sapper project.
#template.svelte
<!--Google Analytics -->
<script async src="https://www.googletagmanager.com/gtag/js?id=UA-SOMEANALYTICSID-1"></script>
<script>
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'UA-SOMEANALYTICSID-1');
</script>
Technically this would be enough for any server-side application but with Sapper thats not the case. All navigation by a user in a Sapper app happens in the client, besides the initial load and page refreshes.
You can ever verify this your self by going to your Google Analytics dashboard in the real-time section. As you can see initial page view does indeed register, so does a page refresh, but not internal navigation with the application.
Don't worry tough we can easily fix that by using the reactive statement I talked about earlier.
Create a Component that Reactively Tracks Client Navigation
Let's create a new component (in your component folder, or where every you see fit).
#components/GoogleAnalytics.svelte
<script>
import { stores } from '@sapper/app';
const { page } = stores();
$: {
if (typeof gtag !== "undefined"){
gtag("config", "UA-SOMEANALYTICSID-1", {
page_path: $page.path
});
}
}
</script>
Here we utilise page stores and the reactive statement and send each new $page.path to Google Analytics upon user navigation.
Load Your Tracking Component in _layout.svelte
Don't forget to load the component in your main _layout.svelte
file.
#routes/_layout.svelte
<script>
import { stores } from '@sapper/app';
import GoogleAnalytics from '../components/GoogleAnalytics.svelte';
import Navbar from '../components/Navbar.svelte';
import Footer from '../components/Footer.svelte';
</script>
<style>
</style>
<GoogleAnalytics />
<Navbar />
<slot></slot>
<Footer />
